Writing a backend server requires field validation when posting data to your database. You can also do that from the front, but what if someone tries to override your frontend validation?
I was learning how to use Laravel to write an API backend server. One of the things I learned was validating the incoming request data. It was pretty easy, and there are many ways to do it, but here’s my favorite way. (Thank you, Shail Gandhi)
To start, make sure you’re on your Laravel project directory and open a terminal to that directory.
In this example, we will create a validation for your blog posts.
Before we finally start, you need to have PHP installed on your machine.
Step 1: Generate a request class file.
Go to your terminal and type in the command below:
php artisan make:request BlogRequest
Make sure the spelling is correct then press enter.
Wait till a success message appears.
This command will create a class file in the Requests directory. From your project’s root directory, go to the app folder, find Http, and go to the Requests folder. There you will find the request class file we just generated. In this example, it is named BlogRequest.php.
Here’s what the file should look like. (It might look different depending on your Laravel’s version but it should be similar).
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class BlogRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return false;
}
/**
* Get the validation rules that apply to the request.
*
* @return array<string, mixed>
*/
public function rules()
{
return [
//
];
}
}
Step 2: Add the Validator and HttpResponseException
We need to use the Validator to validate the requests that the API received and to throw the validator’s errors. To throw the errors, we will need the HttpResponseException.
We will be adding the Validator and HttpResponseException below the use Illuminate\Foundation\Http\FormRequest;
use Illuminate\Foundation\Http\FormRequest;
use Illuminate\Http\Exceptions\HttpResponseException;
use Illuminate\Contracts\Validation\Validator;
If you noticed, there’s an authorized() function. You can use it if you want to authorize your users but in this instance, we will just make it public. I will change the returned value from false to true.
Step 3: Let’s add the rules
As I mentioned earlier, there are a lot of ways to validate requests but writing the rules remains the same. You have to look for the function below.
/**
* Get the validation rules that apply to the request.
*
* @return array<string, mixed>
*/
public function rules()
{
return [
//
];
}
Inside the rules() function, we will write the rules as the function name implies. The format for the returned array is “field_name => ‘RULES_HERE'” like the code below.
return [
"post_title" => 'required|min:5',
"post_content" => 'required|min:10',
];
You can put all the rules next to its name and for multiple rules, use a vertical bar (|). Or you can use array instead, like “post_title” => [“required”, “min:5”].
You can read the documentation for the available rules here: https://laravel.com/docs/9.x/validation#available-validation-rules
Step 4: Send the response
After we validated the request, we would want to send a response if it’s not validated.
Below the rules() function, we will create a new function named “failedValidation(Validator $validator)”. And inside it, we will throw the HttpResponseException as a JSON response like:
public function failedValidation(Validator $validator) {
throw new HttpResponseException(response()->json([
"success" => false,
"message" => "Validation failed!",
"error" => $validator->errors()
]));
}
If you noticed, we used the Validator and the HttpResponseException we used earlier. The Validator will catch all the errors in case the requested data failed to pass the rules we set up. Then we will throw the response using the HttpResponseException. Inside it, you can see the array converted into JSON. The JSON response has a key “error” and inside it, you can see the list of errors.
You are free to change the key and pair values in the response.
Step 5: Let’s use the request in a controller.
At the top, we can add the newly created Request like this:
<?php
namespace App\Http\Controllers;
use App\Http\Requests\BlogRequest;
On the function, you’d like to use the validation, and add/replace the old Request parameters with the new one we created. In my example, it’s BlogRequest so we will write it like this:
public function test(BlogRequest $request) {
return "It worked!";
}
Step 6: Time to test!
Below is the result if the validation failed.
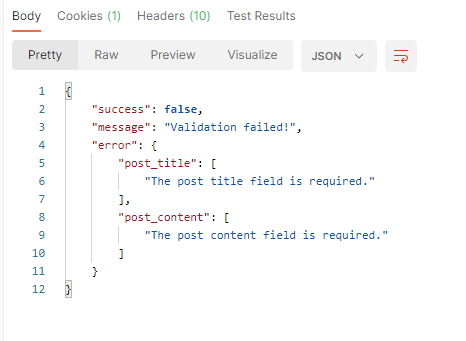
Below is the result of successful validation.
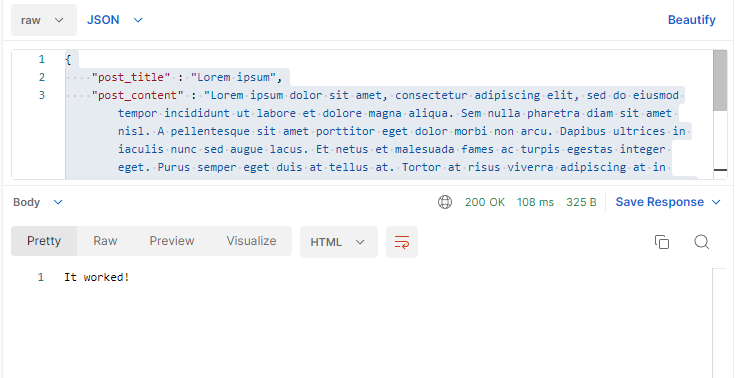
This method is not the only way to do validation for your Requests and you can read more at Laravel’s documentation here: https://laravel.com/docs/9.x
Recent Comments